반응형
https://leetcode.com/problems/valid-parentheses/
Given a string s containing just the characters '(', ')', '{', '}', '[' and ']', determine if the input string is valid.
An input string is valid if:
Open brackets must be closed by the same type of brackets.Open brackets must be closed in the correct order.
Example 1:
Input: s = "()" Output: true
Example 2:
Input: s = "()[]{}" Output: true
Example 3:
Input: s = "(]" Output: false
Constraints:
1 <= s.length <= 104s consists of parentheses only '()[]{}'.
대표적인 스택 문제 입니다. 괄호의 쌍이 모두 맞는지 체크하는 문제로서 난이도는 easy로 매우 쉽습니다. 문자열을 split 함수를 이용하여 모두 분리 시킨 뒤 스택에 문자를 담으면서 전체적인 쌍이 맞는지 체크하면 됩니다.
몇 가지 체크해야 할 점은, 로직이 모두 끝난 뒤 스택이 비어 있는지 체크를 해야 하고, 스택이 비어있는 상태에서 닫는 괄호인 ), ], } 가 오면 그대로 false를 리턴해줍니다.
아래는 소스코드 입니다.
import java.util.*;
class Solution {
public boolean isValid(String s) {
Set<String> opens = Set.of("(", "{", "[");
Stack<String> brackets = new Stack<>();
String[] inputs = s.split("");
for (String bracket : inputs) {
if (opens.contains(bracket)) {
brackets.push(bracket);
continue;
}
if (brackets.empty()) {
return false;
}
String open = brackets.pop();
if (!isPair(open, bracket)) {
return false;
}
}
return brackets.empty();
}
private boolean isPair(String open, String close) {
if (open.equals("(") && close.equals(")")) {
return true;
}
if (open.equals("{") && close.equals("}")) {
return true;
}
if (open.equals("[") && close.equals("]")) {
return true;
}
return false;
}
}
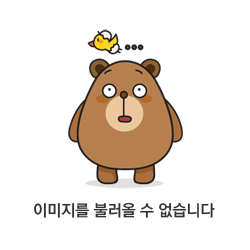
728x90
반응형
'Coding Test' 카테고리의 다른 글
[LeetCode] Number of Islands (0) | 2022.05.16 |
---|---|
[LeetCode] Two Sum (0) | 2022.04.24 |
[프로그래머스] 깊이/너비 우선 탐색(DFS/BFS). 네트워크 (0) | 2022.04.08 |
[프로그래머스] 깊이/너비 우선 탐색(DFS/BFS). 타겟 넘버 (0) | 2022.04.07 |
[프로그래머스] 힙(Heap). 이중우선순위큐 (0) | 2022.03.31 |